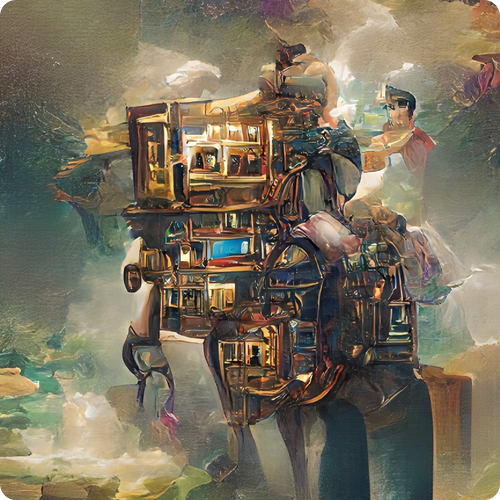
Native JS Transitions
Table of Contents
Easily Create Dynamic Loading Effects
Hello, Explorer! I have a quick tip for you.
Loading animations can be a great way to improve the user experience of your web application. With the following code snippet and CSS classes, you can easily add loading animations to any page.
Whether you apply it to the entire page, or individual elements, this solution can be adapted to fit many scenarios.
TypeScript
First, let’s take a look at the transition
function:
function transition(staggerMs: number): void {
['transition-in-opacity', 'transition-in-left'].forEach((selector) => {
const hiddenSelector = `.${selector}`;
const visibleClass = `${selector}-show`;
let i = 0;
document.querySelectorAll(hiddenSelector).forEach((e: HTMLElement) => {
if (e.style.transitionDelay || e.style.transitionDelay !== '') return;
e.style.transitionDelay = `${i}ms`;
e.classList.add(visibleClass);
i += staggerMs;
setTimeout(() => {
e.style.removeProperty('transition-delay');
e.style.removeProperty('transition-duration');
e.classList.add(visibleClass);
e.classList.remove(showClass);
}, 1000);
});
});
}
This function takes a staggerMs
parameter, which controls the delay between each element’s animation. It iterates over the selectors in the array and applies the corresponding CSS classes to the hidden elements with those selectors. The function also sets the transition-delay property on each element, which creates the staggered effect. A timeout is set to undo the transition delay and duration styles set by the function to prevent future transitions from being effected by loading animations.
CSS
Now let’s take a look at the CSS classes:
.transition-in-opacity {
opacity: 0;
filter: blur(0.05em);
transition: all 0ms;
}
.transition-in-opacity-show {
opacity: 1;
filter: blur(0);
transition: all 2s;
}
.transition-in-opacity-visible {
opacity: 1;
filter: blur(0);
}
.transition-in-left {
opacity: 0;
filter: blur(5px);
transform: translateX(-100%);
transition: all 0ms;
}
.transition-in-left-show {
opacity: 1;
filter: blur(0);
transform: translateX(0);
transition: all 2s;
}
.transition-in-left-visible {
opacity: 1;
filter: blur(0);
transform: translateX(0);
}
These class pairings define the animation styles for the elements targeted by the transition function. Each class has three parts: one for the hidden state (e.g. .transition-in-opacity
), one for the show state (e.g. .transition-in-opacity-show
), and one for the final visible state (e.g. transition-in-opacity-visible
).
The hidden state sets the initial opacity, blur, and transform properties. The show state sets the final opacity, blur, and transform properties, and sets the transition property to all 2s to create a smooth animation effect. The visible state simply removes the transition property as to not impact any future animations.
So, to recap:
.transition-in-opacity
: Sets the initial state for an element to have zero opacity and a slight blur effect.
.transition-in-opacity-show
: When applied, this class will gradually transition the element to full opacity and remove the blur effect over a 2 second duration.
.transition-in-opacity-visible
: Sets the final state for an element to be fully opaque with no blur effect and removes transition effects and delay.
Examples
To use these classes and the transition function in your web application, simply include the CSS classes in your stylesheet and call the transition function with the desired stagger value when you want to animate a set of elements. For example:
<!-- HTML markup for the elements to animate -->
<div class="transition-in-opacity">Element 1</div>
<div class="transition-in-left">Element 2</div>
<!-- JavaScript code to animate the elements -->
<script>
window.addEventListener(
'load',
() => {
transition(100); // animate with a 100ms delay between elements
},
false
);
</script>
Code Pen
See the Pen Native Transitions by Kevin Chatham (@kevinchatham) on CodePen.